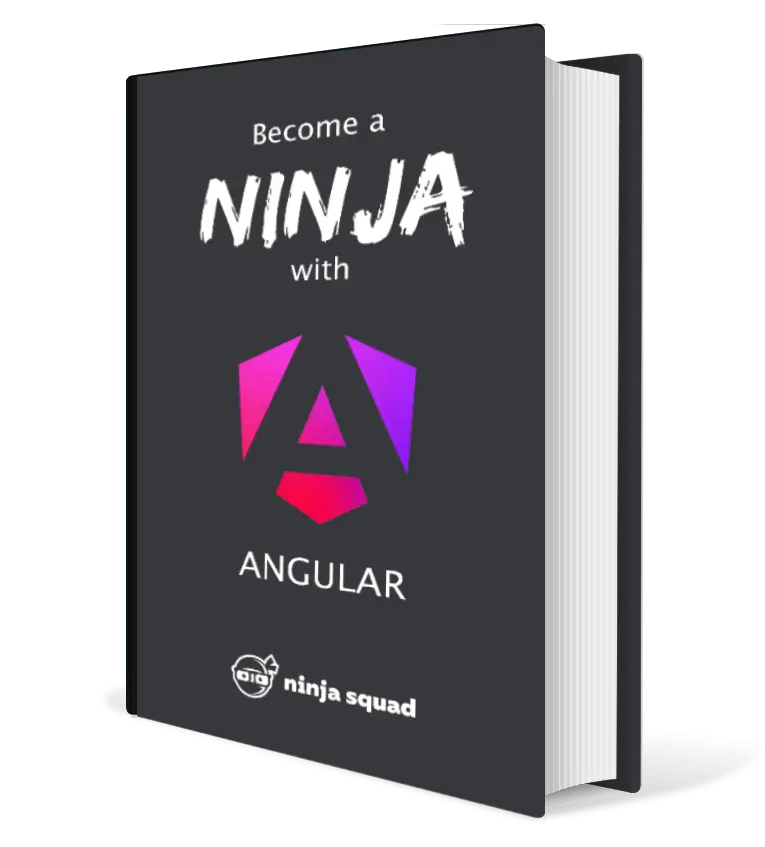
Become a ninja
with Angular
This ebook helps you get the philosophy of Angular, the tools (like ES2015, TypeScript, Angular CLI...), and each part of the framework in a pragmatic way. You will be able to kickstart your project by the end of the reading, and build your amazing apps!
If you want to go further, a Pro Pack is available with exercises to understand each step of building an application. That means tons of pragmatic code samples, tests for each step, good practices for every common use-case. You'll save tens of hours on your next project!
Available in PDF, EPUB & HTML; English & French; Pay with PayPal or card with Stripe.
I've already bought this ebook, I want to download the latest version.
This ebook is regularly updated, see the full changelog. The current version uses Angular 19.2.8.
What you will learn
Discover what we will cover in this book
Modern Angular
The eBook and the exercises, while still mentioning the legacy ways of doing things, focuses on modern Angular: standalone components, reactivity based on signals and also RxJS, and of course TypeScript.
How it works
You will of course discover the syntax of the templates, the dependency injection mechanism, routing, tests, forms, RxJS. But the eBook also explains how the framework works, why some choices are better than others, and the reasons behind design choices.
Best practices
The eBook and the exercises have changed and matured over time, with the evolutions of the framework and thanks to our long experience working on real-life projects. Gain time by benefitting from this experience, gotchas, tips, performance tricks and best practices.
Components
Learn how to best design your pages by dividing them into components, directives and services. Learn the various ways to make them communicate with each other. Integrate ready-to-use libraries into your applications.
Tests
We'll also spend some time talking about automated tests (unit test and end-to-end tests), and learn how to write, execute and maintain them. If you buy the Pro Pack, all the exercises consist in making automated tests pass, and these tests can guide you when writing your owns.
Performance
Performance is at the heart of many architectural choices made by Angular. Transitioning to signals is one of them. You will learn the how, but also the why. And we'll make sure you can write efficient code while not falling into common traps.
Table of contents
Here are all the chapters of our eBook. You can also look at the changelog here.
- IntroductionFree
- A gentle introduction to ECMAScript 2015+Free
- Transpilers
- let
- Constants
- Shorthands in object creation
- Destructuring assignment
- Default parameters and values
- Rest operator
- Classes
- Promises
- Arrow functions
- Async/await
- Sets and Maps
- Template literals
- Modules
- Conclusion
- Going further than ES2015+Free
- Dynamic, static and optional types
- Enters TypeScript
- A practical example with DI
- Diving into TypeScriptFree
- Types as in TypeScript
- Enums
- Return types
- Interfaces
- Optional arguments
- Functions as property
- Classes
- Working with other libraries
- Decorators
- Advanced TypeScriptFree
- readonly
- keyof
- Mapped type
- Union types and type guards
- The wonderful land of Web ComponentsFree
- A brave new world
- Custom elements
- Shadow DOM
- Template
- Frameworks on top of Web Components
- Grasping Angular’s philosophyFree
- From zero to somethingFree
- Node.js and NPM
- Angular CLI
- Application structure
- Our first standalone component
- Bootstrapping the app
- Signals: the building blocks of the application state
- What is a signal?
- Creating, reading and writing signals
- The templating syntax
- Interpolation
- Using other components in our templates
- Property binding
- Events
- Expressions vs statements
- Local variables
- If, For and Switch with the control flow syntax
- Template variables with
@let
- Structural directives
- Template directives
- Summary
- Building components and directives
- Introduction
- Directives
- Selectors
- Inputs with
input()
- The
@Input
decorator - Outputs with
output()
- The
@Output
decorator - Lifecycle
- Component-specific metadata
- Template / Template URL
- Styles / Style URL
- Reacting to signal changes
- Computed signals
- Effects
- Styling components and encapsulation
- Shadow DOM strategy
- Emulated strategy
- None strategy
- Styling the host
- Pipes
- Pied piper
- json
- slice
- keyvalue
- uppercase
- lowercase
- titlecase
- number
- percent
- currency
- date
- async
- A pipe in your code
- Creating your own pipes
- Dependency injection
- DI yourself
- Easy to develop
- Easy to configure
- Other types of provider
- Hierarchical injectors
- DI without types
- Services provided by the framework
- Reactive Programming
- Call me maybe
- RxJS
- Signals and RxJS interoperability
- Testing your app
- The problem with troubleshooting is that trouble shoots back
- Unit tests
- Fake dependencies
- Testing components
- Testing with fake templates, providers…
- Simpler, cleaner unit tests with
ngx-speculoos
- End-to-end tests (e2e)
- Send and receive data through HTTP
- Getting data (
provideHttpClient
) - Transforming data
- Advanced options
- Interceptors
- Context
- Tests
- Getting data (
- Router
- En route (
provideRouter
) - Navigation
- Redirects
- Matching strategy
- Hierarchical and empty-path routes
- Guards
- Resolvers
- Router events
- Parameters and data
- Bind parameters and data to component inputs
- Lazy loading
- En route (
- Forms
- Forms, dear forms
- Template-driven
- Code-driven
- Adding some validation
- Errors and submission
- Add some style
- Creating a custom validator
- Grouping fields
- Reacting to changes
- Updating on blur or on submit only
FormArray
andFormRecord
- Strictly typed forms
- Super simple validation error messages with
ngx-valdemort
- Going further: define custom form inputs with
ControlValueAccessor
- Summary
- Zones and the Angular magic
- ZoneJS
- Change detection
- Angular compilation: Just in Time vs Ahead of Time
- Code generation
- Ahead of Time compilation
- Advanced observables
- Some Like It Hot
- Unsubscriptions
- Automatic unsubscriptions
- Leveraging operators
- Using Subjects as triggers
- Building your own Observable
- Managing state with stores (NgRx, NGXS, Elf and friends)
- Conclusion
- Advanced components and directives
- Input transforms
- View queries:
viewChild
- Content:
ng-content
- Content queries:
contentChild
- Conditional and contextual content projection:
ng-template
andngTemplateOutlet
- Host listener
- Host binding
- DOM manipulation with
afterRender
orafterNextRender
- Angular modules
- A compilation unit
- Module composition
- Functional, routed modules
- Internationalization
- The locale
- Default currency
- Translating text
- Process and tooling
- Translating messages in the code
- Pluralization
- Runtime i18n with Transloco
- Best practices
- Performances
- First load (bundling, compression, lazy-loading, server side rendering)
- Reload (caching, service worker)
- Profiling
- Runtime performances
- Production mode
track
in for loops- Change detection strategies
- Get out of the zone
- Zoneless change detection
- Pure pipes
- Conclusion
- Signals: advanced topics
- Value equality
untracked
- Root and component effects
afterRenderEffect
- Effect cleanup
- Two-way binding with
model
inputs - Linked signals with
linkedSignal
- Async resources with
resource
andrxResource
- HTTP calls with
httpResource
- Deferrable Views with
@defer
@placeholder
,@loading
, and@error
- Conditions
- Prefetching
- How to test deferred loading?
- Going to production
- Environments and configurations
- strictTemplates
- Package your application
- Server configuration
- Conclusion
- This is the end
The Pro Pack
Switch to second gear by building a real project, step by step!
The Pro Pack contains a set of exercises, allowing you to build the PonyRacer app from scratch, confronting yourself to all these common problems in modern JavaScript applications. For each step, you receive a set of instructions corresponding to one of the ebook chapter, and a set of unit and end-to-end tests to validate your code. A NodeJS module we wrote is here to help you analyze your code and compute your score. Your turn to solve the challenge with the help of our tips! When you're done, or if you're stuck, you can check the solution we made. Our platform allows you to follow your progress, and inform you when we update the exercises. You can then switch to this new version (for free), and start again where you stopped. It's a great way to see how the best practices evolve!
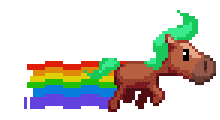
PonyRacer
Build a complete application
Thanks to the Pro Pack, you will create an application to bet on pony races (because everybody loves ponies), but above all, you will learn about all the use-cases you may need in a real Angular application.
Exercises list
Here are all the lessons you can follow with the Pro Pack.
- Getting startedFree
Let's generate the project skeleton, and begin our adventure!
- Templates quizFree
An easy quiz to get started with the templating syntax. Ready?
- TemplatesFree
Learn a bit more about components and the templating syntax with a new component for the responsive menu.
- List of racesFree
Create a new component to display the list of races.
- Race detailFree
Display a more friendly race detail, with a list of ponies.
- Pony componentFree
Add a nice pony component, with a beautiful 8-bit artwork image.
- Using pipesFree
Use a pipe in your template to format raw data.
- Custom pipe with date-fns
Build our own pipe using the third party library date-fns to display pretty dates.
- Race service
Build a service for handling the race business logic, with a hint of Dependency Injection.
- Observables quiz
Let's see how we use RxJS.
- Observables with RxJS
Learn more about reactive programming and use observables in the service.
- HTTP
Fetch data over HTTP from the Ponyracer REST API.
- Router
Have nice URLs that mirror the content displayed using the router.
- Forms quiz
Do you remember how to build forms?
- Login form
Build a simple form to authenticate our users.
- Register form
Build a more complex form with all the Angular goodies.
- Control Value Accessor
Create a custom form component with Control Value Accessor.
- State management
Display our logged in user and add a logout feature with the help of signals.
- Remember me
Having to log in every time is painful: let's remember our user for the next time! Play with the LocalStorage and effects.
- HTTP quiz
Let's check your knowledge of the HTTP API!
- HTTP with authentication
Some REST endpoints are secured: let's see what we need to do to call them, and how to use interceptors. Also a little introduction to JSON Web Token and to how to use environment files.
- Bet on a pony
Where is the fun if we can't bet on a pony? Outputs and RxJS to the rescue!
- Live race
Display the live race with fake data coming from a hand-made observable with RxJS.
- WebSockets
Get real time data from the server over WebSockets!
- Observable tips and tricks
Learn a few handy operators and become a master with RxJS and Observables.
- Boost a pony
We can make a pony much faster by clicking on it: a good excuse to learn some advanced RxJS.
- Reactive user score
Our user score should update in real time. Another use-case for observables and signals!
- Router quiz
Let's check your knowledge of the router!
- Protected routes with guards
Let's use guards to protect parts of our app.
- Nested routes and redirections
A real application often has nested routes and redirections.
- Lazy loading
The router can lazy-load parts of our app. Let's see how!
- Building advanced components
Learn how to build advanced components, using `ng-content`.
- Building advanced directives
Learn how to build advanced directives, using `contentChild`, host listener and host binding.
- Integrate with a UI library
Let's use UI components from ng-bootstrap, a third party library with powerful components.
- Charts in your app
Every professional app has its chart. Let's see how Angular can play with Chart.js.
- Performance tricks
Angular is blazingly fast, but there are still a few tricks to learn to only update the DOM when necessary, and make change detection faster.
- Zoneless application
Let's get rid of zone.js!
- Internationalization
Localization and translations in our application using Transloco.
- Going to production
Final step to deliver our app! What do we need to do to launch our product?
Free samples
Have a look at these free samples to give you an idea.
Pricing and Plans
This book comes in two languages: English and French. The Pro Pack (our online platform full of exercises) can be bought for a team of any size, with an appropriate discount.
eBook | Pro Pack | |
---|---|---|
Pay what you want | €99.00 | |
Buy | Buy | |
ePUB | ||
HTML | ||
English | ||
French | ||
Exercises | ||
Tests | ||
Complete project | ||
Quiz | ||
Source code of the app |
Frequently Asked Questions
Because you always have exotic questions in your mind: we're here to bring you peace with nice answers.
This ebook is for everyone wanting to discover or deepen their knowledge of Angular. Beginner students, experienced developers, absolute fans of other frameworks (Vue.js, React), everyone has found something useful in it.
This ebook can also be a nice gift for a friend! ;)We're test addicts. Every piece of code given as example is statically analyzed and unit tested. While writing this book, we could upgrade with every Angular release and quickly spot every breaking change (and there were lot of them before the beta release...). Thus, you can be assured that every piece of code is working, and that any following Angular release won't break anything. Same thing for the Pro Pack: the project is fully updated and regenerated on every Angular release, with each step automatically tested.
We're writing in Asciidoc, and we're using AsciiDoctor to generate all expected formats (PDF, EPUB, and HTML). A build based on Angular CLI analyzes every sample code, tests it, before embedding it in the ebook source.
Let's be clear: the ebook sales won't pay for the time of its writing, ever (every technical writer will say so).
But we are happy to help, even modestly, for an issue we care about. You can reward the authors for their work, and optionally support charity, whatever the ratio you want.Absolutely not.
No, this is the only restriction: you may not sell it and use it commercially.
We only collect your email, that we need to send you your purchase, your invoice, and an email from time to time to notify you when we update the ebook. You can ask us at any time to delete your email from our database (but we won't be able to send you the updates anymore).
Yes, you'll get an invoice for every purchase. You'll even be able to customize the invoice with your own company details if you need to.
Sure! You just have to input the needed quantity when buying, and you'll get an appropriate discount. Then, you can register your team members one by one, to open their access to the online platform. If you need further explanations, send us an email at hello+books@ninja-squad.com to talk about it.
Thanks for asking! :) Yes, we offer trainings on Angular for every level, but also on other topics that might interest you.
Our support to the EFF
Defend your rights on the Internet while learning Angular!
Ninja Squad has chosen to support this organization during this campaign. When buying our ebook, not only will you decide how much you want to pay, but also how much you want to give to EFF, if you want to. Our company is committed to make regular donations matching your choice.